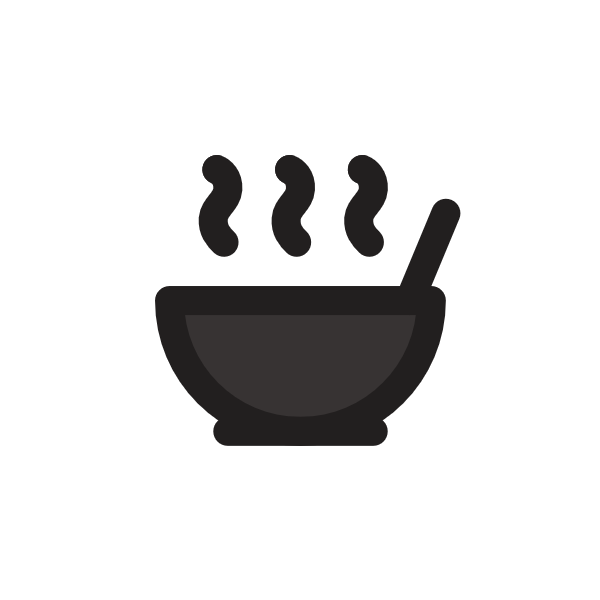
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Timing attack
Read the original article here.
Okay, here is the detailed educational resource on Timing Attacks, framed within the context of "The Forbidden Code: Underground Programming Techniques They Won’t Teach You in School."
The Clock Tells Tales: Understanding and Exploiting Timing Attacks
In the world of information security, the focus is often on cracking the code itself – the mathematical algorithms, the encryption keys, the logical flaws. But sometimes, the deepest secrets aren't hidden within the code, but are whispered by the physical execution of that code. This is where side-channel attacks come into play, and perhaps one of the most elegant and insidious is the timing attack.
Why is this considered "forbidden" knowledge in the traditional sense? Not because it's strictly illegal, but because it delves into the subtle, messy reality of how software interacts with hardware – details often abstracted away in standard programming curricula. It's about exploiting imperfections, variations, and unintended information leakage that arise purely from how long an operation takes. Understanding timing attacks requires thinking like an adversary, looking beyond the intended logic to the underlying physics of computation.
What is a Timing Attack?
Let's start with the core definition:
Timing Attack: A type of side-channel attack where an attacker attempts to compromise a system, often a cryptosystem, by analyzing the time taken to execute specific operations or algorithms.
Think of it like this: If you ask someone to find a specific book on a shelf, and they take longer if the book is at the far end compared to the front, you might be able to guess the book's position just by the time it takes them to find it, even if they never tell you where it was or what the title was. In software, the "secret" data (like a password, a private key bit, or a branch condition) can influence the execution path, the operations performed, or the memory accessed, causing minute, measurable differences in execution time.
The Fundamental Principle: Time is Information
Every single operation a computer performs takes time. A single instruction on a CPU, a memory lookup, a mathematical calculation – they all consume clock cycles. Crucially, the time taken for these operations can vary based on the input data.
For example:
- Comparing two strings might stop early if the first characters don't match, or continue longer if they match for many characters.
- Multiplying two numbers might take a different number of clock cycles depending on the specific values and the CPU architecture.
- Accessing data from the CPU cache is vastly faster than accessing it from main memory, and whether data is in the cache depends on what data was recently accessed (which might be influenced by secret inputs).
By precisely measuring the time it takes for a target system (like a web server performing cryptographic operations or a login system checking a password) to respond to different inputs, an attacker can work backward. They analyze the timing variations and correlate them with their inputs to infer information about the system's internal state or the secret data being processed.
This can be significantly easier than traditional cryptanalysis, which often requires complex mathematical analysis of ciphertext without any leakage from the execution process. Timing information provides a completely separate, often unintended, channel of leakage. In some cases, timing data might not reveal the whole secret directly but can narrow down the possibilities, making traditional attacks or brute-force attempts much more feasible.
Why Does Time Vary Based on Data? The Micro-Architecture Whispers
The theoretical execution time of an algorithm might be constant for a given input size, but the actual time on a real CPU is anything but constant. This variation is often data-dependent and stems from low-level hardware and implementation details:
Memory Access Patterns (Cache Timing):
- CPUs use caches – small, very fast memory areas that store copies of frequently used data from main memory.
- Accessing data already in the cache (cache hit) is extremely fast (a few clock cycles).
- Accessing data not in the cache (cache miss) is much slower, requiring fetching it from main memory (dozens or hundreds of clock cycles).
- If a secret value determines which data is accessed, an attacker observing whether a memory access results in a hit or a miss via timing can learn something about the secret. This is a fundamental mechanism exploited in modern attacks like Meltdown and Spectre.
Conditional Jumps and Branch Prediction:
- Code with
if
,else
,while
, orfor
loops often involves conditional jumps (changing the flow of execution based on a condition). - Modern CPUs use branch prediction to guess which path a conditional jump will take before the condition is fully evaluated. This keeps the pipeline full and speeds up execution.
- If the prediction is correct, execution continues smoothly.
- If the prediction is incorrect, the CPU has to discard the work it did down the wrong path and restart down the correct one. This results in a significant, measurable delay (misprediction penalty).
- If a secret value influences the condition of a branch, an attacker might be able to infer the value by observing if a misprediction penalty occurs for specific inputs. This is why secure code often aims to be branch-free when dealing with secrets.
- Code with
Variable-Time CPU Instructions:
- Some complex operations on certain CPU architectures do not take a fixed amount of time.
- Integer Division: Often implemented using microcode loops that might take fewer steps for small divisors or dividends.
- Bit Shifts and Rotations: On older CPUs without a barrel shifter, shifting/rotating
N
bits might involve repeating a 1-bit shiftN
times. IfN
is secret, the time varies linearly withN
. - Multiplication: Similar to division on older architectures.
- While modern CPUs have constant-time implementations for many basic operations, relying on this across all architectures and versions can be risky.
Examples from the Battlefield
Timing attacks are not theoretical curiosities; they have been successfully demonstrated and exploited against real-world systems.
Cryptographic Key Recovery (RSA, DSA, ElGamal):
- Many cryptographic algorithms, particularly those involving modular exponentiation (like
base^exponent mod modulus
), use techniques like the "square-and-multiply" algorithm. - The steps performed in square-and-multiply depend on the individual bits of the exponent (the private key). A '1' bit might involve both a square and a multiply, while a '0' bit only involves a square.
- If the multiplication operation takes measurably longer than the squaring operation (due to hardware or implementation), the total execution time will subtly vary based on the number or position of '1' bits in the secret key.
- An attacker can perform many operations with the same key but different public inputs, measure the time for each, and use statistical analysis to correlate timing variations with the key bits, eventually recovering the entire key.
- Many cryptographic algorithms, particularly those involving modular exponentiation (like
Exploiting Implementation Flaws (SSL/TLS with RSA and CRT):
- A famous attack by Boneh and Brumley in 2003 targeted SSL-enabled web servers. It didn't exploit a flaw in the RSA algorithm itself but in a common optimization using the Chinese Remainder Theorem (CRT) for faster decryption.
- The specific implementation details of the CRT optimization on certain platforms resulted in timing variations based on the private key during decryption.
- Despite network latency adding noise, they were able to collect enough timing samples over a network connection to statistically recover a server's private key in hours.
- This led to the widespread adoption of blinding countermeasures in SSL/TLS implementations, adding random noise or modifying inputs to decorrelate timing from the secret key.
Leaking Non-Cryptographic Secrets (Unix Login):
- In older versions of Unix, the
login
program would first check if the entered username was valid before hashing the entered password using the computationally expensivecrypt
function. - If the username was invalid, the
crypt
function (which took seconds on older hardware) was skipped. The login attempt finished quickly. - If the username was valid, the
crypt
function was called. The login attempt took measurably longer (seconds). - An attacker could simply time login attempts. Short times indicated an invalid username; long times indicated a valid one. This allowed them to build a list of valid usernames without needing passwords, greatly simplifying brute-force attacks.
- The fix: Always call the
crypt
function with a dummy value if the username is invalid, ensuring the timing is constant regardless of username validity.
- In older versions of Unix, the
Cross-Process Communication and Attacks (Cache/VM attacks, Meltdown/Spectre):
- Even securely isolated processes on the same system can interact via shared resources like CPU caches or virtual memory paging.
- A malicious process can manipulate the cache/memory state and then time the operations of a victim process. If the victim process's operations depend on secret data which affects its cache/memory access patterns, the attacker can infer information about the secret by observing their own access times to probes in the cache/memory.
- The infamous Meltdown and Spectre attacks (2017/2018) leveraged speculative execution (a form of branch prediction) combined with cache timing attacks. They tricked the CPU into speculatively accessing protected memory locations and, based on the secret data found there, perform operations that left timing traces in the cache. The attacker could then measure the cache access times to recover the forbidden data, even though the speculative execution was later rolled back.
Code Case Study: Insecure vs. Timing-Safe String Comparison
A classic and easily understood example is comparing two strings, especially when one is a secret (like a password or a cryptographic key component).
Here's a typical, seemingly innocent, but timing-insecure comparison function, similar to memcmp()
in C:
int insecure_compare(const unsigned char *a, const unsigned char *b, size_t len) {
size_t i;
for (i = 0; i < len; ++i) {
if (a[i] != b[i]) {
// EXPLOITABLE POINT: Returns early if characters mismatch!
// Time taken depends on the index 'i' of the first difference.
return (a[i] < b[i]) ? -1 : 1;
}
}
// Returns 0 only if all characters match
return 0;
}
Explanation of Vulnerability: If an attacker is trying to guess a password P
of length N
, and they provide a guess G
.
- If
G
matchesP
for the firstk
characters but differs atk+1
, the loop runsk+1
times. - If a different guess
G'
matchesP
for the firstk+1
characters but differs atk+2
, the loop runsk+2
times. - By measuring the execution time, the attacker can determine how many leading characters of their guess match the secret password. This allows them to guess the password one character at a time much faster than brute-forcing the entire string. Guessing a password of length
N
becomes roughlyN * C
attempts (whereC
is the size of the character set) instead ofC^N
attempts.
Here's a timing-safe comparison function:
int timing_safe_compare(const unsigned char *a, const unsigned char *b, size_t len) {
size_t i;
volatile unsigned char result = 0; // 'volatile' helps prevent some compiler optimizations
for (i = 0; i < len; ++i) {
// Use bitwise XOR to check inequality
// OR the result into the accumulator.
// This check happens for *every* character regardless of previous results.
result |= a[i] ^ b[i];
}
// The result will be non-zero if any pair of bytes were different.
// It will be zero ONLY if all pairs were identical.
// Time taken depends ONLY on 'len', the length of the strings, NOT the content.
return result;
}
Explanation of Countermeasure: This function always loops exactly len
times. It calculates the XOR difference for every pair of characters. The |=
operation accumulates whether any difference was found. The final result check result == 0
only happens after the loop finishes. The time taken is primarily dictated by the loop iterations (len
) and constant operations within the loop, making it much less dependent on the content of the strings being compared.
Libraries like OpenSSL (CRYPTO_memcmp
) and libsodium (sodium_memcmp
) provide such constant-time comparison functions specifically for sensitive data like cryptographic keys or passwords.
Countermeasures: Building Timing-Safe Systems
Preventing timing attacks requires a mindset shift from just correctness to constant-time execution when handling secrets.
Constant-Time Algorithm/Implementation: An algorithm or its specific implementation is considered constant-time (or timing-safe) if its execution time does not vary based on the value of secret inputs, but only perhaps on the size of the inputs.
Achieving timing safety is difficult and requires careful attention at multiple levels:
- Algorithmic Design: Choose algorithms that are inherently constant-time or can be implemented that way.
- Implementation Practices:
- Avoid Data-Dependent Branches: Rewrite code to avoid
if
statements or loops whose conditions or iteration counts depend on secret data. Use bitwise operations and conditional moves instead where possible. - Use Constant-Time Arithmetic: Be aware of CPU instructions (like division, shifts) that might have variable timing and use constant-time alternatives or ensure the variable parameter is not secret.
- Constant-Time Memory Access: Ensure memory access patterns (which data is read/written) do not depend on secret values, or use techniques like pre-fetching or always reading the same amount of data to smooth out cache timing differences.
- Avoid Data-Dependent Branches: Rewrite code to avoid
- Blinding: For some cryptographic operations (like RSA decryption), add random noise to the input before processing and remove it from the output afterward. This makes the timing variations less correlated with the actual secret key.
- Padding and Masking: Process fixed-size blocks or use masking techniques to hide the actual value of the secret being operated on.
- Careful Testing: Even with careful coding, subtle timing leaks can remain. Specialized tools and statistical analysis are needed to verify that an implementation is truly timing-safe.
It's important to note that constant-time implementations often mean doing the "worst-case" amount of work every time, which can incur a performance penalty. This is a necessary trade-off for security.
Limitations and Practical Challenges for the Attacker
While powerful, timing attacks are not always easy to execute:
- Noise: Real-world systems are noisy. Network latency, operating system multitasking, disk access, background processes, and hardware interrupts introduce random variations into timing measurements. An attacker needs to collect many, many samples (sometimes millions or billions) and use statistical methods to average out the noise and find the signal.
- Measurement Accuracy: Obtaining high-resolution timing measurements can be challenging, especially remotely over a network or when restricted by the operating system's timer resolution.
- Implementation Knowledge: Effective timing attacks often require the attacker to have some knowledge (or make educated guesses) about the target system's hardware, operating system, algorithm, and specific implementation details to understand where the timing variations might occur. However, as Kerckhoffs's Principle and Shannon's Maxim remind us ("The enemy knows the system"), security should not rely on the obscurity of the implementation. Attackers can often reverse-engineer or obtain similar hardware to profile its timing characteristics. Timing attacks can even be used to help identify the algorithm or implementation in use.
The "Forbidden" Takeaway
Timing attacks represent a class of vulnerabilities that bridge the gap between theoretical security and practical implementation flaws. They highlight that perfect security requires not just robust algorithms but also meticulous attention to the physical execution environment.
For those exploring "the forbidden code," understanding timing attacks is crucial because:
- It reveals how subtle side channels can completely undermine otherwise strong cryptographic protections.
- It requires a deep understanding of how software executes on hardware, down to cache behavior and CPU micro-operations.
- It demonstrates the power of statistical analysis and signal processing in cybersecurity.
- It equips you to build more robust, timing-safe code yourself, anticipating potential leakages.
Ignoring timing vulnerabilities is a common mistake in software development, making this area a fertile ground for advanced exploitation and a critical skill for anyone serious about deep system security. The clock is always ticking, and for the skilled adversary, its rhythm can reveal the most guarded secrets.